If Else Conditional Statement in Java
If Else Conditional Statement in Java.In this article we will step into how to create an if else statement in JAVA. Generally speaking all programming Languages has its own version of if else statement in their Language.
Basically the if else statement are logical statement use by machine to return an action under some specific condition.For instance , if this condition happen , what is the action need to be taken, else if another condition take place what possible action need to be taken. Is fairly logical.
During my University years, i used to hate programming a lot.Why is that so? At that time, I felt overwhelming with all the programming parameter , syntax’s, flows etc. The real problem which make me hate programming so much, is that i did not received any good guidance from my school or lecturer , books on how to learn things. Remember those thick programming books which beat around the bush , that does not go straight to the point in explanation , and the Lecturer as well populating the white board with notes , that hardly explain how things works.
That imprint fear within me latter in life , when any thing in relation come to programming. Thanks to all the Thick yellow pages programming books and confusing university lectures which do not actually know what they are doing.I learn things through the hard way without guidance or help . I hope someone would have thought me how to learn things in an easy way at that time.
The things that you most fear of in life , are the things that you must conquer.So if you fear of programming conquer it now,before it is too late.
Lets Step into how to create an If Else Conditional Statement in Java referring the code below.
In this Example , i will create a BMI Calculator using an If Else Statement.
Under this BMI Class , two Methods will be created. The First function will accept the input height and weight from the user .
In this Sample we will skip the checking of wrong input type by the User, as this will need Exception Error Handling to do the Job
The second method will received the BMI Calculated from the first function.
- First Create a BMI Class in Java.
- Import java.util.Scanner; as we are going to take in the user input later below in the programme .
- Create 3 variable in doubleto accept the input data from the User in the programme later below
- Create 2 new Scanner Object name inputscan1 and inputscan2.to take in the value of weight and height
- Now we need to create the First Method calledbmiCaculator(double weight,double height) this method will accept the Height and weight parameter from the user.
- Create the Formula to calculate the BMI . in this case is the BMI Formula is weight divided square of height.
- When the function finish calculate the value. Return the value into bmiRatio variable
- Then the next step is to create a Void Method that does not return any value called compareBMI which take in the value calculated by the bmiCaculator and compare the value and categorise whether the user is under weight , just good , overweight or obese.
- At every single Condition it will print out the status of the USER.
- Now we have finished creating both function lets go back to main and called both of our function
- First print out the Statement to ask the user to input his or her weight
- Take the user input weight through inputScan1 and assign the user input value into static double inputWeight;
- Same thing applied to the User input height
- Notified the User Calculated BMI by calling the Function,.System.out.println(“Your BMI is”+bmiCaculator(inputWeight,inputHeight));
- comparebmi= bmiCaculator(inputWeight,inputHeight);Pass the Calculated BMI Function to static double comparebmi;
- Pass the comparebmiinto the compareBMI() method
- The User will be able to see a print out of his / her health status
package oop2; import java.util.Scanner; public class Bmi { static double inputWeight; static double inputHeight; static double comparebmi; public static void main(String[] args) { Scanner inputScan1 = new Scanner(System.in); Scanner inputscan2 =new Scanner(System.in); System.out.println("Key in your Weight ?"); System.out.println(""); inputWeight= inputScan1.nextDouble(); System.out.println("Key in your Height ?"); System.out.println(""); inputHeight= inputscan2.nextDouble(); System.out.println("Your BMI is"+bmiCaculator(inputWeight,inputHeight)); System.out.println(""); comparebmi= bmiCaculator(inputWeight,inputHeight); System.out.println(" "); compareBMI(comparebmi); } public static double bmiCaculator(double weight,double height){ double bmiRatio = 0; double heightSquare = Math.pow(height, 2); bmiRatio = weight/heightSquare; return bmiRatio; } public static void compareBMI(double inputBMI){ if(inputBMI<18.5){ System.out.println("You are under weight"); } else if(inputBMI>=18.5 && inputBMI<=24.9 ){ System.out.println("You are pretty Healthy yay"); } else if(inputBMI>=25 && inputBMI<=29.9 ){ System.out.println("You are overweight!"); } else { System.out.println("You are obeses!"); } } }
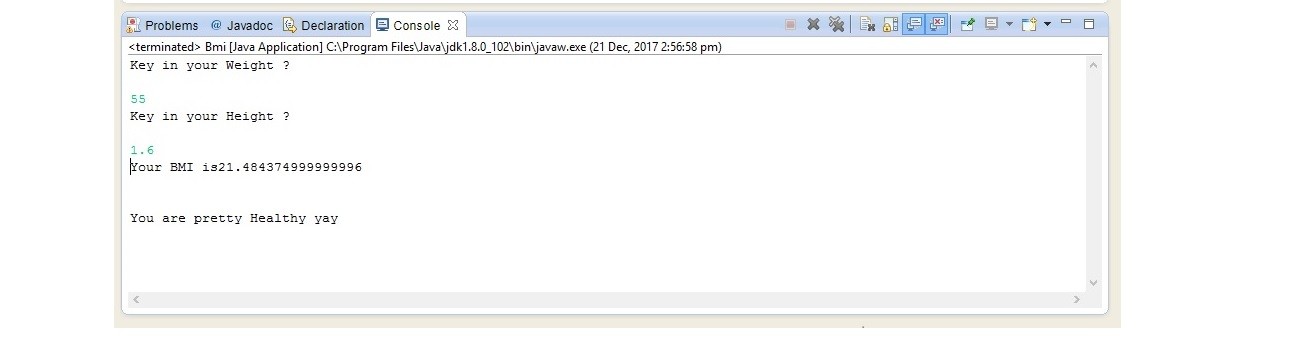
Well this wrap up the article about If Else Conditional Statement in Java.
Check out Oracle site here
Check out how to create constructor in Java here
Leave a Reply